28.Strings in Python
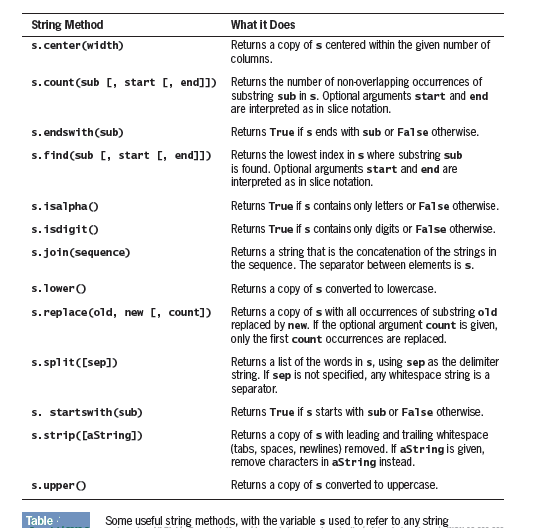
Strings are compound data type made of sequence of characters. Hence from the string, the individual charters can also be directly accessed. Strings are created by using single quotes or double quotes or triple quotes. Example: >>>s1="Python Programming" >>>s2='Python Programs' >>>s3="""Python is a powerful Programming language""" This will create a string object s1, s2 and s3 using different forms.Individual characters can be accessed using different subscripts( +ve or –ve) with the string object. The positions of string's characters are numbered from 0.So the 5th character is in position 4. When working with strings, the programmer sometimes must be aware of a string's length.Python len() function can be used to find the number of characters in the string Eg: >>> len('python') 6 >>> len("") 0 Note: The string is an immutable data structure.This means that its inter...